The N76E003AT20 is a low-power, high-speed microcontroller from the N76E003 family designed and developed by Nuvoton. It is an 8-bit unit based on 8051 architecture MCU, with a total of 20 pins, of which 18 pins are GPIO pins, peripherals, and memory. It comes with a powerful oscillator of 16MHz, SPI connector, I2C protocol, self-wake-up timer, an A/D converter (ADC) with 12-bit resolution, PWM with 8-channels, 18K flash memory and a fault brake for motor controlling operation. These microcontrollers are used in small application projects and consumer products. The Keil u-Vision IDE and C51 compiler is used to program this Nuvoton microcontroller unit. This article explains the block diagram and ADC peripheral with programming code to read the analog voltage of the N76E003AT20 microcontroller interfacing and its working.
How to Interface ADC Peripheral with the N76E003AT20 Microcontroller:
One of the most common features of the microcontroller hardware is the ADCs (Analog to Digital Converters), which convert the analog voltage to a digital voltage value. Because the microcontrollers are digital devices and operated with binary values (1’s and 0’s). Since they cannot process given analog data directly. Therefore, the ADC is utilized to obtain an analog voltage and convert it to a digital voltage value equivalent that the microcontroller understands. Due to this in-built ADC feature of the N76003AT20 microcontroller, different sensors with analog output can be interfaced.
In this section, let’s know how to use the in-built ADC peripheral feature of the N76003AT20 microcontroller. Here, a small voltage divider circuit with a potentiometer is used. To read and display the analog voltage ranging from 0V to 5V, a 16×2 LCD is used. In this project, the analog input pins of the microcontroller are used to read the analog voltage. The components required for this application are;
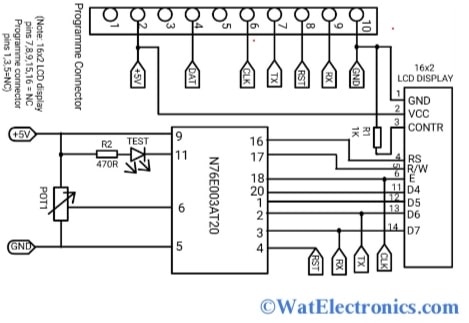
Interfacing Diagram ADC Peripheral Of N76E003AT20
- N76E003AT20 microcontroller development board with Nu-link programmer
- 5V power supply
- 16×2 LCD Display
- Resistor – 1K
- Trim pot or potentiometer 50K
- Few bergs and hook-up wires
- Breadboard
From the pin configuration, the port P0 has the maximum number of analog pins, which are used for communication-related to the LCD. From the above diagram, the GPIO pins port P0 pins of the N76E003AT20 microcontroller like Pin16 -(P0.0/PWM3/IC3/MOSI/T1), pin17-(P0.1/PWM4/IC4/MISO), pin 18 (P0.2/ICPK/OCDCK/RXD_1[SCL]); pin20-(P0.4/AIN5/STADC/PWM3/IC3), pin1- (P0.5/PWM2/IC6/T0/AIN4), pin2-(P0.6/TXD/AIN3), pin3-(P0.7/RXD/AIN2) pins)] are used to connect with 16×2 LCD pins like Pin 4 – RS, pin 5 – R/W, pin 6 – E, pin 11 – D4, pin 12 -D5, pin 13 – D6, and pin 14 -D7 pins.
The D4, D5, D6, and D7 data pins of the 16×2 LCD are used to display the value of the ADC. Here, P3.0 and P1.7 can be used as analog input pins AIN1 and AIN0. But only one analog input pin P1.7/AIN0 (Analog Input channel 0) is required for this project. The POT1 potentiometer is connected to the P1.7 analog input channel 0 pins as shown in the diagram that works as a voltage divider network and its reading is monitored and written by this AIN0 (Analog Input Channel 0). The programmer connector is for the interfacing programming connection. Use the DC adapter for the power supply and the LCD to read the output voltage on the port pin P1.7 (AIN0 pin), which is set by using the potentiometer.
The N76E003AT20 microcontroller has a 12-bit Successive Approximation Register (SAR) ADC feature with better resolution and provides 8 input channels in single-end mode. Therefore interfacing ADC with this microcontroller is very easy and simple. Firstly, select and set the ADC channel input or the I/O pins for the direct mode in the code. Since the analog input channel pins are the input pins, they can be set only in the high impedance mode (Only-Input mode) by using PxM2 and PxM1 registers.
To interface, the 16×2 character LCD with the N76E003AT20 microcontroller download the library and include the LCD.h and lcd.c files in the N76E003 Keil application project. The display related function given in the library are as follows,
- Initializing the 16×2 LCD
- The command is sent to the 16×2 LCD.
- Writing to the 16×2 LCD
- Enter a string on the 16×2 LCD.
- Print a character, by passing in its hexadecimal value.
- Scroll messages longer than 16 characters.
- Directly print whole numbers to the 16×2 LCD.
The coding of ADC in the N76E003AT20 microcontroller interfacing to read the analog voltage on the AN0 pin is given below.
Code
#include “N76E003.h”
#include “SFR_Macro.h”
#include “Function_define.h”
#include “Common.h”
#include “Delay.h”
#include “lcd.h”
#define bit_to_voltage_ratio 0.001220703125 // 5.0V divided by 4096 For 12-Bit ADC
void setup (void);
unsigned int ADC_read(void);
float voltage;
char str_voltage[20];
void main(void){
int adc_data;
setup();
lcd_com (0x01);
while(1){
lcd_com (0x01);
lcd_com (0x80);
lcd_puts(“ADC Data: “);
adc_data = ADC_read();
lcd_print_number(adc_data);
voltage = adc_data * bit_to_voltage_ratio;
sprintf( str_voltage, “Volt: %0.2fV”, voltage);
lcd_com(0xC0);
lcd_puts(str_voltage);
Timer0_Delay1ms(500);
}
}
void setup (void){
Set_All_GPIO_Quasi_Mode;
lcd_init();
Enable_ADC_AIN0;
lcd_com (0x80);
LCD_ScrollMessage(“Welcome “);
lcd_com (0x80);
lcd_puts(“ADC interfacing”);
lcd_com (0xC0);
lcd_puts (“With N76E003 mcu”);
Timer3_Delay100ms(5);
}
unsigned int ADC_read(void){
register unsigned int adc_value = 0x0000;
clr_ADCF;
set_ADCS;
while(ADCF == 0);
adc_value = ADCRH;
adc_value <<= 4;
adc_value |= ADCRL;
return adc_value;
}
Explanation:
- The Enable_ADC_AIN0 setup function sets the AIN0 input pin of the ADC and configures the registers ADCCON1, ADCCON0 and AINDIDS.
- Then the registers ADCRH and ADCRL will read the ADC value with a resolution of 12-bit.
- Then the bits for 4 times are shifted left and added to the data variable. In its main function, the ADC reads the data and displays it on the LCD screen directly. However, voltages are also converted using voltage ratios or voltage values divided by bit values. A 12-bit ADC provides 4095 bits at an input voltage of 5.0V. (That means 5.0V / 4095 = 0.001221V, a small change by 1 bit is approximately equal to a change by 0.001V).
- The function sprintf is used for converting the data from the bit value to the voltage value.
Working:
When the potentiometer POT1 is turned or varied, the voltage applied to the ADC pin (AIN0 pin) also changes. Then the ADC value and the analog voltage value will be displayed on the 16×2 character LCD. In this way, the ADC peripheral in the N76E003AT20 microcontroller interfacing is used to read the analog voltage on the analog channel input pin.
Please refer to this link to know more about N76E003AT20 Microcontroller Datasheet.
Thus, this is all about an overview of the block diagram and programming ADC peripheral of the N76E003AT20 microcontroller. The fault brake in this microcontroller is implemented in fusion with the PWM circuit enhancement used to protect the motor from damage by providing the fault detection input. This MCU is widely used in battery chargers, access control systems, thermostats, Bluetooth speakers, small home appliances, data collection systems, electric meters, motor control, industrial applications, etc. Check the datasheet link given above to understand how to control the Brushless DC motor with the Nuvoton N76003AT20 microcontroller.