RTC or Real-Time Clock is a timekeeping device that is used in various time-critical devices like GPS, data loggers & servers. Generally, RTC modules are available with a battery setup where it keeps the RTC module running when external power is not available. This module maintains the Data and Time up to date. So RTC module provides a precise time and date when we required it. There are various RTC modules available like DS3221, DS1307 & DS3231 where these modules include an oscillator, controller & an embedded quartz crystal resonator. So this article discusses an overview of the DS3231 RTC module, interfacing with the Arduino board and its applications.
What is DS3231 RTC Module?
The DS3231 module is an I2C real-time clock with an integrated temperature-compensated crystal oscillator & crystal. Once the power of this module is interrupted, then the device has input from the battery so that this module maintains a precise time. The long-term precision of this module can be enhanced by the crystal oscillator. The RTC module maintains the time like secs, minutes, hrs, days, years, months & dates. The RTC module has an AM or PM indication which works either in 12hrs or 24hrs mode.
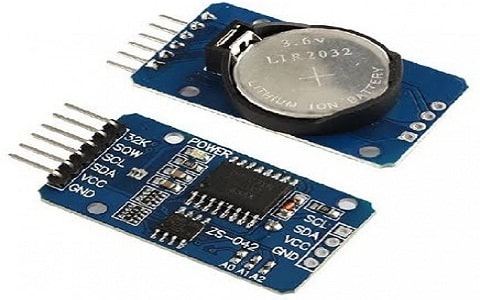
DS3231 RTC Module
This DS3231 is one of the most frequently used RTC modules that track the current date & time. So this module is used in different electronics projects for maintaining time and date. For precise timekeeping, this module includes the AT24C32 EEPROM. In addition, this module provides precise timekeeping results for a long time because of the existence of the crystal resonator.
DS3231 RTC Module Pin Configuration:
DS3231 RTC module includes different pins where each pin and its function are discussed below.
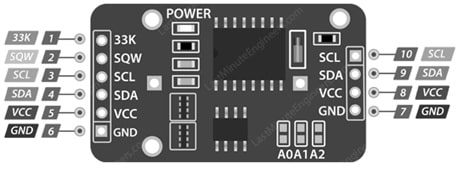
DS3231 RTC Module Pin Configuration
- 33K Pin: This is the o/p pin that provides the compensated temperature & precise reference CLK.
- SQW Pin: This pin at different frequencies provides a square wave and also an interrupt signal.
- SCL Pin: This pin is the serial CLK pin which is mainly used within I2C communication.
- SDA Pin: This is the serial data pin, utilized in I2C communication.
- VCC Pin: This is the module’s power supply pin, so connect it through 3.3V/5V
- GND Pin: This GND pin is mainly used to provide common GND.
Features & Specifications:
The features & specifications of the DS3231 RTC module include the following.
- This module functions with low voltages.
- A pushbutton for resetting time.
- It includes a battery backup.
- A two-directional and400 kHz of I2C interface mainly for speedy transmission.
- EEPROM – 32 bytes to read/write & save data.
- RTC module is used in two modes either 12hrs/24hrs format.
- It automatically switches from a power source to an integral battery source.
- A programmable Square-wave o/p as per necessity.
- It maintains the information of secs, mins, hrs, days, weeks, months & years.
- Type of package is 16, 300-mil SO package.
- Operating temperature ranges from -45 to -800C
- Max voltage (VCC) is +0.3 Volts.
- The accuracy of temperature is 30C.
- Operating voltage ranges from 2.3 to 5.5 V.
- The battery backup current is 500 mA.
- Accuracy @-40 – 800C is ±3.5 ppm.
- Accuracy @ 0 – 400C is ± 2.0 ppm.
Interfacing RTC DS3231 with Arduino UNO Board
This RTC module is used to maintain the updated track of time because it calculates the passage of time, so used in nearly every electronic device. It maintains the complete track of data of seconds, minutes, hours, dates, months, days, and so on. The DS3231RTC CLK simply works in two formats either in 24 hrs or 12 hrs. The RTC module is similar to the system clock but the main dissimilarity is that the RTC module works even once the power of the system is OFF whereas the system CLK cannot function in that manner.
This RTC module includes a backup battery, so it allows the module to keep the time and date even once the power is not available. So, you don’t require retuning the power repeatedly. This module is very precise, and economical and works on either 3.3V/5V. RTC DS3231 modules use the 12C communication protocol, so it simply utilizes two Arduino board pins which are very possible for an Arduino.
The interfacing of RTC DS3231 with the Arduino UNO board is shown below. The required components for this interfacing mainly include; RTC DS3231 Module, Arduino UNO, USB Cable Type A to B, and Jumper Wires.
The connections of this interfacing follow as;
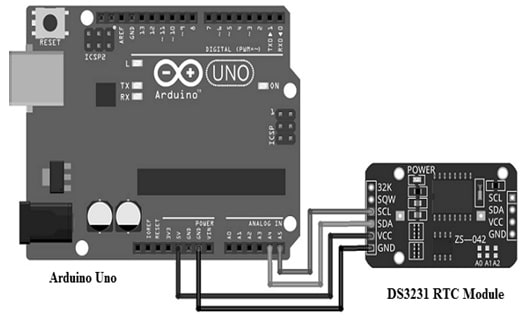
Interfacing RTC DS3231 with Arduino UNO Board
The A5 pin of Arduino Uno is connected to the SCL pin of the RTC module.
The A4 pin of Arduino Uno is connected to the SDA pin of the RTC module.
The 5V pin of Arduino Uno is connected to the VCC pin of the RTC module.
The GND pin of Arduino Uno is connected to the GND pin of the RTC module.
Code
#include “Wire.h”
#define DS3231_I2C_ADDRESS 0x68
// Convert normal decimal numbers to binary coded decimal
byte decToBcd(byte val){
return( (val/10*16) + (val%10) );
}
// Convert binary coded decimal to normal decimal numbers
byte bcdToDec(byte val){
return( (val/16*10) + (val%16) );
}
void setup(){
Wire.begin();
Serial.begin(9600);
// set the initial time here:
// DS3231 seconds, minutes, hours, day, date, month, year
setDS3231time(40,7,16,5,6,6,22);
}
void setDS3231time(byte second, byte minute, byte hour, byte dayOfWeek, byte
dayOfMonth, byte month, byte year)
{
// sets time and date data to DS3231
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set next input to start at the seconds register
Wire.write(decToBcd(second)); // set seconds
Wire.write(decToBcd(minute)); // set minutes
Wire.write(decToBcd(hour)); // set hours
Wire.write(decToBcd(dayOfWeek)); // set day of week (1=Sunday, 7=Saturday)
Wire.write(decToBcd(dayOfMonth)); // set date (1 to 31)
Wire.write(decToBcd(month)); // set month
Wire.write(decToBcd(year)); // set year (0 to 99)
Wire.endTransmission();
}
void readDS3231time(byte *second,
byte *minute,
byte *hour,
byte *dayOfWeek,
byte *dayOfMonth,
byte *month,
byte *year){
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set DS3231 register pointer to 00h
Wire.endTransmission();
Wire.requestFrom(DS3231_I2C_ADDRESS, 7);
// request seven bytes of data from DS3231 starting from register 00h
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f);
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
void displayTime(){
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
// retrieve data from DS3231
readDS3231time(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month,
&year);
// send it to the serial monitor
Serial.print(hour, DEC);
// convert the byte variable to a decimal number when displayed
Serial.print(“:”);
if (minute<10){
Serial.print(“0”);
}
Serial.print(minute, DEC);
Serial.print(“:”);
if (second<10){
Serial.print(“0″);
}
Serial.print(second, DEC);
Serial.print(” “);
Serial.print(dayOfMonth, DEC);
Serial.print(“/”);
Serial.print(month, DEC);
Serial.print(“/”);
Serial.print(year, DEC);
Serial.print(” Day of week: “);
switch(dayOfWeek){
case 1:
Serial.println(“Sunday”);
break;
case 2:
Serial.println(“Monday”);
break;
case 3:
Serial.println(“Tuesday”);
break;
case 4:
Serial.println(“Wednesday”);
break;
case 5:
Serial.println(“Thursday”);
break;
case 6:
Serial.println(“Friday”);
break;
case 7:
Serial.println(“Saturday”);
break;
}
}
void loop(){
displayTime(); // display the real-time clock data on the Serial Monitor,
delay(1000); // every second
}
The above code first includes the library for the RTC module. In addition, it has formulated the program to change the numbers from decimal to binary & binary to decimal. For this reason, it is used as the variable byte.
In the void setup, it has set the early time. Set all the different parameters like sec, min, hr, date, date, month & year. After that, the code is multiplied by all these parameters through the byte variable. After that, it has the code that permits fetching the data from the RTC module. Additionally, it transmits it to the serial monitor. In the void loop, it simply used the function of display time to display the RTC.
Connect the DS3231 RTC Module through the Arduino UNO board. Once the connections are made, copy the above code & paste it into your Arduino IDE. After that, upload this code to the Arduino board and open the serial monitor. Now you can observe the present time & date. The CLK would work even once you separate the power from the Arduino board, it won’t get rearranged.
Applications
The applications of the DS3231 RTC module include the following.
- This module is used where you need precise times & dates like laptops, computers, mobiles, embedded system-based application devices, etc.
- This RTC module uses extremely less power to work, thus this module is used on mobile systems.
- It is used wherever maximum speed communication is required.
- This module can also function on both 12Hr & 24Hr formats in GPS systems.
- These modules are used in gaming, robotics, utility power meters, computer peripherals, GPS, etc.
- This module is used in various time-critical applications & devices like servers, data loggers, etc.
- This is a low-cost real-time CLK that is normally used with microcontrollers to keep track of the world’s Time & Date.
- The DS3231 RTC module is used in different electronic devices which uses clock like computers, laptops, watches, etc.
- These modules are used to make different DIY, robotics, Arduino, electronics, electrical, and Raspberry Pi projects.
- These RTC modules are used in servers, data loggers, GPS modules, power meters, etc.
Please refer to this link for N76E003AT20 Microcontroller, DS3231 RTC Module Datasheet.
Thus, this is an overview of the DS3231 RTC module – pin configuration, specifications, features, interfacing, and its applications. The RTC DS3231 Module allows precise time-keeping. This module includes an in-built 32 kHz crystal oscillator, temperature sensor & alarm CLK capability. This RTC module utilizes a typical I2C interface & can be interfaced easily with different development boards. Here is a question for you, what are the other RTC modules available in the market?