The waterproof digital temperature sensor is a one-meter-long sealed & pre-wired probe. This sensor is handy whenever you have to measure something distant, or in damp conditions. These are digital sensors, so you don’t receive any signal degradation even above long distances. This sensor is fairly precise and provides up to 12 bits of precision from the onboard DAC. So they work great with any microcontroller through a single digital pin. Thus, this sensor uses the Dallas 1-Wire protocol only which is fairly complex and needs a bunch of code to analyze the communication. This article elaborates on the DS18B20 temperature sensor, its working, and its applications.
What is the DS18B20 Temperature Sensor?
The DS18B20 is a single-wire waterproof digital temperature sensor invented by Dallas Semiconductor. So it is available in Stainless-steel probe form and has characteristics like high precision, small size, strong anti-interference ability, low hardware overhead, etc. Thus, this temperature sensor is ideal for measuring temperature in different conditions, such as underwater, wet conditions, something far away, or under the ground.
This temperature sensor measures a wide temperature range, from -55°C to +125°C (-67°F to +257°F), with +-5% accuracy. So, this sensor uses a single-wire bus protocol to communicate with a microcontroller and transmit the temperature readings. Thus, its resolution ranges from 9 bits to 12 bits, but the default resolution to power up the temperature sensor is 0.0625°C precision, i.e., 12 bits.
How DS18B20 Temperature Sensor Work?
The DS18B20 waterproof temperature sensor works similarly to a temperature sensor. So it measures temperature and ADC conversion with a convert-T command. So the output temperature value can be stored in the 2-byte register in the sensor and later, it returns to its stationary state. This temperature sensor includes three wires or pins like Vcc, GND & data wires mainly for operation.
DS18B20 digital temperature sensor provides temperature measurements that range from 9-bit to 12-bit Celsius. Thus this sensor can also have an alarm function including nonvolatile user-programmable lower and upper trigger points. This sensor communicates above a 1-Wire bus that needs one data line only for communication through a central microprocessor. So this sensor additionally derives power straight from the data line by removing the need for an exterior power supply.
So every DS18B20 sensor includes a unique 64-bit serial code that allows several DS18B20 sensors to work on a similar 1-Wire bus. Thus, it is very easy to utilize one microprocessor for controlling several sensors that are distributed above a large area.
DS18B20 Pin Configuration:
The DS18B20 temperature sensor pin configuration is shown below. So this sensor includes three pins which are explained below.
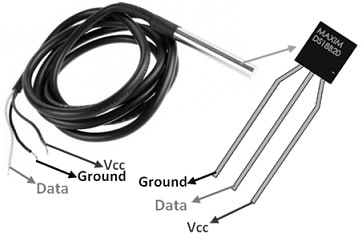
DS18B20 Pin Configuration
- Pin-1 (GND): This is the Ground pin of the temperature sensor, so connected to the microcontroller’s GND terminal.
- Pin-2 (VCC): This is the power supply pin of the temperature sensor so connected to the 3.3V/5V terminal of the microprocessor or microcontroller.
- Pin-3 (DATA): This is the o/p pin of the temperature sensor that provides the output with one wire method. So it must be attached to a digital pin on the microprocessor or microcontroller.
Features & Specifications:
The features and specifications of the DS18B20 temperature sensor include the following.
- DS18B20 is a digital temperature sensor.
- This sensor is available in different packages like 8-Pin SO, 8-Pin µSOP & 3-Pin TO-92.
- Its unique 64-bit address allows multiplexing.
- It doesn’t need external components.
- This sensor needs two pins only for operation in parasitic power mode.
- Several temperature sensors can share a single pin of microprocessor/microcontroller.
- It includes programmable alarm options.
- Its unique single-wire interface needs a single digital pin of microprocessor or microcontroller only for its communication.
- Its accuracy is ±0.5°C
- This sensor’s resolution is 9- bits, 10- bits, 11- bits, (or) 12-bits.
- Its operating voltage ranges from 3V to 5.5V.
- DS18B20 sensor includes an alarm feature with low and high user-settable temperature thresholds
- These sensors are waterproof, so used in moisture-exposed environments.
- It communicates through a 1-Wire bus that requires only one data line used for communication.
- Each DS18B20 sensor has a unique 64-bit serial code that allows several DS18B20 sensors to work on a similar 1-Wire bus.
Equivalents & Alternatives
DS18B20 temperature sensor equivalent is DS18S20. So alternative temperature sensors are; TMP100, Thermocouple, LM75, SHT15, DHT11, TPA81, LM35DZ, D6T, etc.
PIC16F877A Interfacing with DS18B20 Temperature Sensor
The DS18B20 temperature sensor interfaces with the PIC16F877A, as shown below. So, interfacing the PIC16F877A microcontroller with the temperature sensor is straightforward, allowing the system to display temperature readings on a 16×2 LCD screen.
Thus, DS18B20 is a three-pin temperature sensor from analog devices that utilizes a single-wire protocol to communicate with a microcontroller or microprocessor. So each DS18B20 sensor has a unique 64-bit serial code that allows various DS18B20 sensors to work on the similar 1-Wire bus which is controlled through one master device. Thus, this sensor provides from 9-bit to 12-bit Celsius temperature measurement resolution
The required components to make this interfacing mainly include; a PIC16F877A microcontroller, DS18B20 temperature sensor, 16×2 LCD screen, 8 MHz crystal oscillator, 22pF ceramic capacitors, 4.7k & 10K ohm resistors, 5 VDC power source, breadboard and jumper wires.
Thus the connections of this interfacing follow as follows;
Connect the DS18B20 sensor data pin to the RB1 pin of PIC16F877A.
Connect the microcontroller’s PORTD pins to the 16×2 LCD module.
Here, the sensor reads the temperature value and displays it on the LCD.
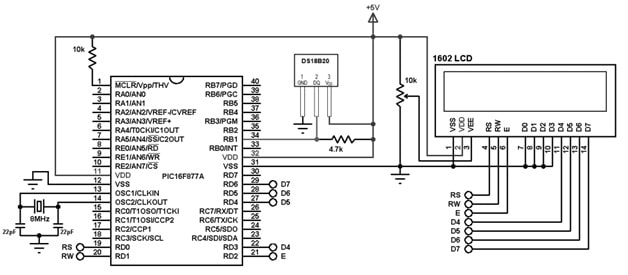
PIC16F877A Interfacing with DS18B20 Temperature Sensor
Code
Below, we present the required code for interfacing the PIC16F877A with the DS18B20 temperature sensor.
#define LCD_RS_PIN PIN_D0, LCD_RW_PIN PIN_D1, LCD_ENABLE_PIN PIN_D2, LCD_DATA4 PIN_D3;
#define LCD_DATA5 PIN_D4, LCD_DATA6 PIN_D5, LCD_DATA7 PIN_D6;
//End LCD module connections
#include <16F877A.h>
#fuses HS, NOWDT, NOPROTECT, NOLVP
#use delay(clock = 8MHz)
#include <lcd.c>
#define DS18B20_PIN PIN_B1
signed int16 raw_temp;
float temp;
int1 ds18b20_start(){
output_low(DS18B20_PIN); // Send reset pulse to the DS18B20 sensor
output_drive(DS18B20_PIN); // Configure DS18B20_PIN pin as output
delay_us(500); // Wait 500 us
output_float(DS18B20_PIN);
delay_us(100); //wait to read the DS18B20 sensor response
if (!input(DS18B20_PIN)) {
delay_us(400); // Wait 400 us
return TRUE; // DS18B20 sensor is present
}
return FALSE;
}
void ds18b20_write_bit(int1 value){
output_low(DS18B20_PIN);
output_drive(DS18B20_PIN);
delay_us(2); // Wait 2 us
output_bit(DS18B20_PIN, value);
delay_us(80); // Wait 80 us
output_float(DS18B20_PIN); // Configure DS18B20_PIN pin as input
delay_us(2); // Wait 2 us
}
void ds18b20_write_byte(int8 value){
int8 i;
for(i = 0; i < 8; i++)
ds18b20_write_bit(bit_test(value, i));
}
int1 ds18b20_read_bit(void) {
int1 value;
output_low(DS18B20_PIN);
output_drive(DS18B20_PIN); // Configure DS18B20_PIN pin as output
delay_us(2);
output_float(DS18B20_PIN);
delay_us(5); // Wait 5 us
value = input(DS18B20_PIN);
delay_us(100);
return value;
}
int8 ds18b20_read_byte(void) {
int8 i, value = 0;
for(i = 0; i < 8; i++)
shift_right(&value, 1, ds18b20_read_bit());
return value;
}
int1 ds18b20_read(int16 *raw_temp_value) {
if (!ds18b20_start()) /
return FALSE;
ds18b20_write_byte(0xCC); // Send skip ROM command
ds18b20_write_byte(0x44);
while(ds18b20_read_byte() == 0); // Wait for conversion complete
if (!ds18b20_start())
return FALSE; // Return 0 if error
ds18b20_write_byte(0xCC); // Send skip ROM command
ds18b20_write_byte(0xBE);
*raw_temp_value = ds18b20_read_byte(); // Read temperature LSB byte and store it on raw_temp_value LSB byte
*raw_temp_value |= (int16)(ds18b20_read_byte()) << 8; // Read temperature MSB byte and store it on raw_temp_value MSB byte
return TRUE; // OK –> return 1
}
void main() {
lcd_init(); // Initialize LCD module
lcd_putc(‘\f’);
lcd_gotoxy(3, 1); // Go to column 3 row 1
printf(lcd_putc, “Temperature:”);
while(TRUE) {
if(ds18b20_read(&raw_temp)) {
temp = (float)raw_temp / 16;
lcd_gotoxy(5, 2); // Go to column 5 row 2
printf(lcd_putc, “%f”, temp);
lcd_putc(223);
lcd_putc(“C “); // Print ‘C ‘
}
else {
lcd_gotoxy(5, 2); // Go to column 5 row 2
printf(lcd_putc, ” Error! “);
}
delay_ms(1000); // Wait 1 second
}
}
// End of code.
Working
There are different functions utilized within the above code which are explained below.
int1 ds18b20_start() function is used to identify whether the temperature sensor is connected properly or not to the circuit. So if the connection is OK then it returns TRUE (1) or else it returns to FALSE (0) when an error occurs.
Void ds18b20_write_bit(int1 value) function sends a single bit to the sensor so the bit is ‘value’ which may be 0 or 1.
The void ds18b20_write_byte(int8 value) function writes a single byte to the sensor, so this function mainly depends on the prior function that writes LSB first.
int1 ds18b20_read_bit(void) function reads a single bit from the sensor and thus returns the read value either 1 (or) 0.
int8 ds18b20_read_byte(void) function reads 1 byte from the sensor so it is based on the earlier function that reads LSB first.
int1 ds18b20_read(int16 *raw_temp_value) function reads the temperature raw data i.e 16-bit long, so the data can be stored within the variable raw_temp_value and it returns TRUE if it is OK and returns to FALSE if any error occurs.
The temperature value in degrees Celsius is equivalent to the raw value so it is separated by 16. So this sensor’s default resolution is 12 bits.
Thus, the remaining program is described throughout the mentioned comments.
Advantages & Disadvantages
The advantages of the DS18B20 temperature sensor include the following.
- DS18B20 temperature sensors are small in size.
- These are durable.
- It has a metal covering that protects the sensor from heat & water.
- Multiple sensors can be connected on a similar bus.
- This sensor is easily integrated into a variety of systems.
- It has a user-configurable resolution.
- It provides precise and reliable temperature data for different applications.
The disadvantages of the DS18B20 temperature sensor include the following.
- This temperature sensor is expensive.
- It takes much time to complete a reading.
- It may not be available with comprehensive packaging, thus you may require using external circuits to work it.
- Data transmission delay occurs.
- Its temperature range is limited ranges from -55°C to 125°C.
Applications
The applications of the DS18B20 temperature sensor include the following.
- The DS18B20 temperature sensor measures the temperature within rigid environments like soil, chemical solutions, mines, and more.
- Users apply it to measure liquid temperature.
- Industries employ it in thermostat control systems as a temperature-measuring device.
- This sensor finds use wherever individuals need to calculate temperature at several points.
- Industrial systems utilize this sensor to measure the temperature of machinery, processes, equipment, and so on.
- Manufacturers use it in consumer products to measure temperature.
- HVAC systems utilize it to measure temperature within buildings, mainly for their controls.
- Thermometers, thermostatic controls, thermally sensitive systems, harsh environments, and Hydro-projects use this temperature sensor.
- Industries use this sensor for temperature measurement, environmental sensing, liquid temperature monitoring, weather stations, and HVAC environmental controls.
Please refer to this link for the DS18B20 temperature sensor Datasheet.
Thus, this is an overview of the DS18B20 temperature sensor, pinout, features, specifications, interfacing, working, pros, cons, and its uses. Thus, this temperature sensor can accurately measure temperatures and serves different applications like aquariums, HVAC systems, weather monitoring, etc. Here is a question for you, what is a DHT11 sensor?